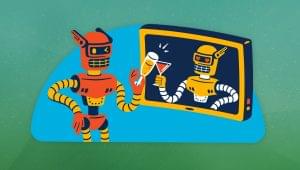
In this quick JavaScript regex matching guide, you'll learn how to test if a string matches a regular expression using the test() method.
In this quick JavaScript regex matching guide, you'll learn how to test if a string matches a regular expression using the test() method.
Learn how to use Math.random to generate random numbers in JavaScript and create random colors, letters, strings, phrases, passwords, & more.
Learn how to transform the character case of a string — to uppercase, lowercase, and title case — using native JavaScript methods.
Learn about methods for rounding numbers in JavaScript: rounding up, rounding down, rounding to decimal places, and gotchas to avoid.
Learn how to split a string into substrings in JavaScript with both the substring() and split() methods, and how to choose which one to use.
Learn how to convert numbers to ordinals in JavaScript. Getting the ordinal of a number allows you to display it in a human-readable format.
Learn three simple ways to convert a string into a number in JavaScript, with simple-to-follow examples and tips on which method to use.
Learn four simple ways to convert a number into a string in JavaScript, with simple-to-follow examples and tips on which method to use.
The REST API is a key part of web infrastructure. Learn about REST and REST APIs, and how web apps communicate over HTTP like web browsers and servers do.
In this TypeScript vs JavaScript comparison, you'll learn about TypeScript's advantages and disadvantages, and when and when not to use it.
Follow this tutorial to build your own Wordle alternative for numbers in JavaScript: Numble.
Nanny State helps you manage state simply and efficiently in JavaScript without Redux, Vuex or other large libraries
Learn about the browser's global object — the window object — which represents the browser window that contains a web page.
Learn about the most used and useful APIs built in to the standard Node.js runtime to save you time and improve your app's efficiency.
The best JavaScript charting libraries for creating and graphs and figures. From the scientific to the simple.
In this guide, we will show you how you can learn to code with JavaScript for free. The JavaScript programming language is versatile, popular, and in high demand — making it a great language for learning how to code.
Learning always takes more time than we'd like. If you’re learning JavaScript, these six mental tricks will help you get there faster.
Learn when to use function expressions vs. function declarations in JavaScript, and how these methods for defining functions are different.
Learn how to use JavaScript arrow functions, understand fat and concise arrow syntax, and what to be aware of when using them in your code.
Higher-order functions can take other functions as arguments or return a function as a result. Learn how to use them and why they're useful.
Learn how to take your first steps with Svelte, a relatively new JavaScript frontend framework for developing websites and web apps.
Learn about for...in loops in JavaScript: their syntax, how they work, when to use them, when not to use them, and what you can use instead.
The micro frontend approach splits apps into small, independent pieces. Learn five pitfalls of using this architecture and how to avoid them.
Learn what Google's zx library is, how to use it, and how it helps make shell scripting with Node.js much more efficient and enjoyable.
A UI library can help kickstart your React app or web dev project. Learn about 9 popular React UI component libraries and when to use them.
Learn how easy it is to create a beautiful, interactive map with HTML, CSS & Leaflet, a user-friendly, open-source JavaScript mapping library.
A micro frontend approach lets us split a frontend app into small, independent pieces. Learn five key reasons why this approach is so useful.
Learn the basics of using Vue Router, such as router setup, passing custom parameters, page navigation, and implementing a 404 page.
Learn how Hasura and PostgreSQL can help you speed up app development and launch backends quickly with minimal effort.
Learn how to deploy serverless functions alongside a front-end app and create an API that generates images and grabs metadata from links.